Tutoriels Backend
HttpClient with angular
We will add the HttpClient mechanism to our project to communicate with an HTTP server.
We will be using the Angular javascript framework version 18.0.2
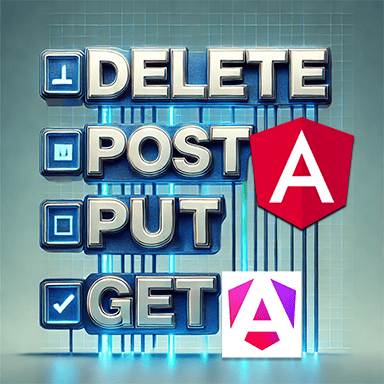
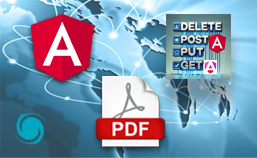
If you don't have time to read this entire guide,
download it now
What are we going to do?
This is step 9 of ourAngular guide which will allow us to obtain a PWA type Web Application .
The basic Angular project we will be using already has the following features
- Generated with Angular CLI
- The Routing
- Lazy Loading
- The Bootstrap CSS Framework
- Server Side Rendering
- Progressive Web App
- Search Engine Optimization
All the sources created are indicated at the end of the tutorial.
The application is at the following address
Installation
Our Angular application represents our Frontend part.
We will use Angular's Httpclient module to access a REST API that will represent our Backend part.
The purpose of this tutorial is not to develop a backend, so we will arbitrarily choose an API available on the web.
JSONPlaceholder is a free REST API ideal for tutorials.
It is accessible with the following link https://jsonplaceholder.typicode.com/
The steps will be as follows:
- Create an items module
- Change the visual interface of the frontend
- Perform routing
- Adapt the module to call the REST API
Let's start by creating our Items module, this being specific to our application will be created in the modules/application directory.
# Creation of the items module
ng generate component pages/application/example-items/items --flat --module=app
ng generate module pages/application/example-items/items --flat --routing
# Creating the items module (Method 2)
ng g c pages/application/example-items/items --flat --module=app
ng g m pages/application/example-items/items --flat --routing
The pages/ application/example-items directory is automatically created by Angular CLI
Let's modify the following files
- app.routes.ts
- items.components.ts
- items-routing.module.ts
- items.module.ts
{
path: 'httpclient',
loadChildren: () => import('./pages/application/example-items/items.module')
.then(mod => mod.ItemsModule)
},
import { Component } from '@angular/core';
@Component({
selector: 'app-items',
templateUrl: './items.component.html',
styleUrl: './items.component.css'
})
export class ItemsComponent {
}
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { ItemsComponent } from './items.component';
const routes: Routes = [{ path: '', component: ItemsComponent }];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class ItemsRoutingModule {}
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { ItemsComponent } from './items.component';
import { ItemsRoutingModule } from './items-routing.module';
@NgModule({
imports: [CommonModule, ItemsRoutingModule],
declarations: [ItemsComponent],
exports: [ItemsComponent]
})
export class ItemsModule {}
npm run start
Then type the url
http://localhost:4200/httpclient
Integration-01
All that remains is to integrate the httpclient management into our Items section.
To respect Angular best practices all features will be added in the app/modules/items directory.
We will create a service to manage access to the api.
The steps are as follows.
- Create a service via an items.service.ts file
- Edit the items.service.ts file to access the api
- Modify the items.component.ts file to call the service
- Modify the items.module.ts file to call the necessary angular module
- Edit the items.component.spec.ts and items.service.spec.ts test files
- Edit the items.component.html file to customize the display of the result
- Edit the home.component.ts file to allow linking with httpclient
Let's start by creating the service with the corresponding angular-cli command and then modify the mentioned files.
# Creation of the items service
ng generate service pages/application/example-items/items --flat
# Creating the items service (Method 2)
ng g s pages/application/example-items/items --flat
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs';
const httpOptions = {
headers: new HttpHeaders(
{
'Content-Type': 'application/json',
}
)
};
@Injectable({
providedIn: 'root'
})
export class ItemsService {
constructor(private http: HttpClient) { }
getItems(url: string): Observable<object> {
return this.http.get(url, httpOptions);
}
}
import { Component, OnInit } from '@angular/core';
import { ItemsService } from './items.service';
import { isPlatformBrowser } from '@angular/common';
import { PLATFORM_ID, APP_ID, Inject } from '@angular/core';
@Component({
selector: 'app-items',
templateUrl: './items.component.html',
styleUrls: ['./items.component.css']
})
export class ItemsComponent implements OnInit {
// eslint-disable-next-line @typescript-eslint/no-explicit-any
items: any;
loaded: boolean;
constructor(
@Inject(PLATFORM_ID) private platformId: object,
@Inject(APP_ID) private appId: string,
private ItemsService: ItemsService
) {
this.loaded = false;
}
ngOnInit(): void {
this.getUsers();
}
getUsers(): void {
this.loaded = false;
this.ItemsService.getItems('https://jsonplaceholder.typicode.com/users')
.subscribe(
items => {
this.items = items;
this.loaded = true;
const platform = isPlatformBrowser(this.platformId) ?
'in the browser' : 'on the server';
console.log(`getUsers : Running ${platform} with appId=${this.appId}`);
});
}
resetUsers(): void {
this.items = null;
this.loaded = true;
}
}
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { ItemsComponent } from './items.component';
import { ItemsRoutingModule } from './items-routing.module';
import { ItemsService } from './items.service';
@NgModule({
imports: [CommonModule, ItemsRoutingModule],
declarations: [ItemsComponent],
exports: [ItemsComponent],
providers: [ItemsService]
})
export class ItemsModule {}
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { HttpClientModule } from '@angular/common/http';
import { ItemsComponent } from './items.component';
describe('ItemsComponent', () => {
let component: ItemsComponent;
let fixture: ComponentFixture<ItemsComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
imports: [
HttpClientModule
],
declarations: [ItemsComponent]
}).compileComponents();
});
beforeEach(() => {
fixture = TestBed.createComponent(ItemsComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
import { TestBed } from '@angular/core/testing';
import { HttpClientModule } from '@angular/common/http';
import { ItemsService } from './items.service';
describe('ItemsService', () => {
let service: ItemsService;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [
HttpClientModule
]
});
service = TestBed.inject(ItemsService);
});
it('should be created', () => {
expect(service).toBeTruthy();
});
});
<div class="row pb-4">
<div class="col-12 col-sm-12 col-md-3 col-lg-3 col-xl-3">
<div class="row pb-4">
<div class="card" style="width: 18rem;">
<div class="card-body">
<h5 class="card-title">Feature : HttpClient</h5>
<hr>
<p class="card-text">Use HtppClient</p>
<button type="button" class="btn btn-primary mr-4" (click)="getUsers()">Get</button>
<button type="button" class="btn btn-primary" (click)="resetUsers()">Reset</button>
</div>
</div>
<div *ngIf="!loaded">
<div class="spinner-grow text-primary" role="status">
<span class="sr-only">Loading...</span>
</div>
<div class="spinner-grow text-secondary" role="status">
<span class="sr-only">Loading...</span>
</div>
<div class="spinner-grow text-success" role="status">
<span class="sr-only">Loading...</span>
</div>
<div class="spinner-grow text-danger" role="status">
<span class="sr-only">Loading...</span>
</div>
<div class="spinner-grow text-warning" role="status">
<span class="sr-only">Loading...</span>
</div>
<div class="spinner-grow text-info" role="status">
<span class="sr-only">Loading...</span>
</div>
<div class="spinner-grow text-dark" role="status">
<span class="sr-only">Loading...</span>
</div>
</div>
</div>
</div>
<div class="col-12 col-sm-12 col-md-8 col-lg-8 col-xl-8">
<div class="row">
<div class="table-responsive httpclient-table blue-gradient" *ngIf="loaded">
<table class="table table-hover table-striped table-responsive-md">
<thead>
<tr>
<th scope="col">name</th>
<th scope="col">username</th>
<th scope="col">email</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of items">
<td>{{item.name}}</td>
<td>{{item.username}}</td>
<td>{{item.email}}</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
.httpclient-table {
border-radius: 10px;
}
.httpclient-table table {
color: #FFFFFF;
}
.httpclient-table.blue-gradient {
background: linear-gradient(40deg, #45cafc, #5664bd) !important
}
{
name: 'Items',
description: 'Items',
icon: 'fab fa-bootstrap',
link: 'httpclient'
},
Errors
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { ItemsComponent } from './items.component';
import { ItemsRoutingModule } from './items-routing.module';
import { ItemsService } from './items.service';
@NgModule({
imports: [CommonModule, ItemsRoutingModule],
declarations: [ItemsComponent],
exports: [ItemsComponent],
providers: [ItemsService]
})
export class ItemsModule {}
import { ApplicationConfig, isDevMode } from '@angular/core';
import { provideRouter } from '@angular/router';
import { HttpClientModule } from '@angular/common/http';
import { importProvidersFrom } from '@angular/core';
import { routes } from './app.routes';
import { provideClientHydration } from '@angular/platform-browser';
import { provideServiceWorker } from '@angular/service-worker';
export const appConfig: ApplicationConfig = {
providers: [
importProvidersFrom(HttpClientModule),
provideRouter(routes),
provideClientHydration(),
provideServiceWorker('ngsw-worker.js', {
enabled: !isDevMode(),
registrationStrategy: 'registerWhenStable:30000'
})]
};
Conclusion
# Development
npm run start
http://localhost:4200/
# Tests
npm run lint
npm run test
# AOT compilation
npm run build
# SSR compilation
npm run build:ssr
npm run serve:ssr
http://localhost:4000/
How to create a From scratch application?
Create your ganatan account
Download your complete guides for free
Démarrez avec angular CLI 
Gérez le routing 
Appliquez le Lazy loading 
Intégrez Bootstrap 
Utilisez Python avec Angular 
Utilisez Django avec Angular 
Utilisez Flask avec Angular 
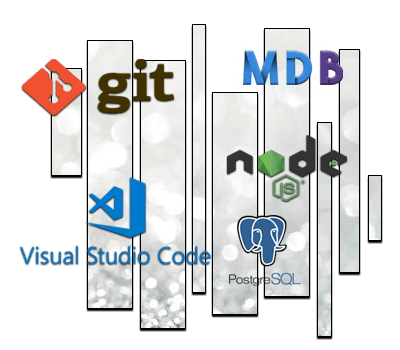