Understanding Modules with Angular 18
Angular is a Typescript framework that allows you to manage the notion of modules in two ways.
The first using ECMAScript 6 modules and the second using NgModules, the Angular specific system.
These are two different but complementary features, which allow us to better structure the code of our applications.
In this comprehensive guide we will see how to use modules with Javascript and Angular NgModules .
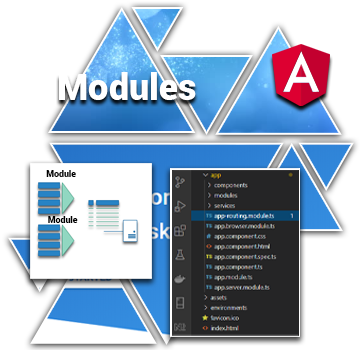
What we are going to do
- Origin of modules
Why and how and modules were integrated into the Javascript language?
- Javascript and modules
How to use modules with JavaScript through a simple example.
- Angular CLI and NgModules
How to use NgModules and integrate modules with Angular.
- Creating a project From scratch
We will use Angular CLI to create a test application from scratch.
- Using our prototype project
We will use an existing project containing the essential functionality.
The project was generated with Angular CLI.
It uses Routing and Lazy Loading.
It integrates the Bootstrap CSS Framework.
- Routing and modules
How interpolation works.
- Lazy loading and modules
How to manage Angular architecture using Angular CLI?
- Perform the Tests
We will test our application via unit and end-to-end testing integrated into Angular.
- Source code
The complete project code on github.
Origin of modules
To answer this question, let's go back for a few moments to the history of computing.
If you want to create web applications, you need to use a computer .
If you want to communicate with a computer, the easiest way is to speak the same computer language (or Programming language in English).
JavaScript is one of these languages.
It is precisely a scripting programming language .
It was invented in 1995 by Brendan Eich to create interactive web pages.
A set of standards have been invented to handle script-like programming languages.
This standard is called ECMAScript .
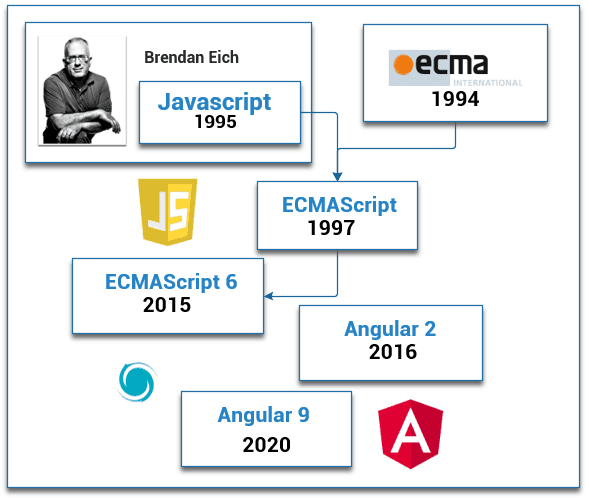
ECMA (for European Computer Manufacturers Association ) is a standardization organization.
It was created in 1961 to standardize computer systems in Europe.
It has been called Ecma International since 1994.
Over the years different ECMAScript versions have been published, among these we could cite
- Version 1: 1997
- Version 2: 1998
- Version 6: 2015
- Version 7: 2016
- Version 10: 2019
The version that will interest us in particular is version 6.
It is more commonly called ES6 or ES2015 .
- ES6 for ECMAScript Edition 6
- ES2015 for ECMAScript Edition 2015
The reason for our interest.
Introducing many concepts like promises, classes, iterators, generators.
And above all what is the subject of this tutorial.
Modules
Why use modules?
Over the past ten years, web applications have become increasingly complex.
The JavaScript programs that allow you to create these applications thus become more complex and therefore larger.
To overcome these difficulties, it was necessary to create a mechanism allowing JavaScript programs to be divided into several parts called modules .
With the modules it could come down to this.
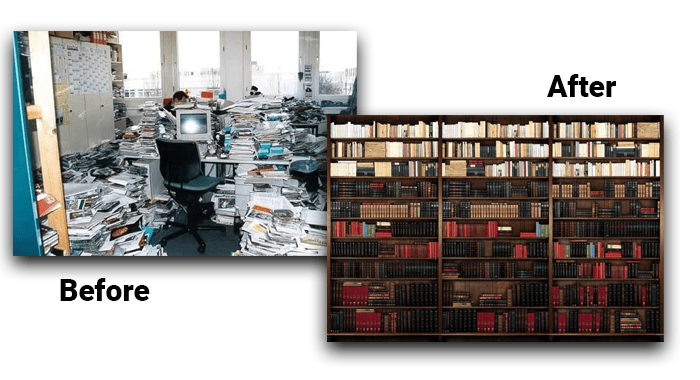
The advantages include
- Structure our applications (several files instead of just one).
- Reuse code.
- Encapsulate code and make it easier to control.
- Manage dependencies more easily.
The use of modules in JavaScript relies on two statements
- import
- export
In JavaScript, modules are simple files containing JavaScript code.
To activate an if module just write the import statement.
This syntax of ES6 modules corresponds to a construction standard recommended in the ECMAScript specifications of the javascript language.
A small code example below.
# The code to export a module
export class AppComponent { ... }
# The code to import a module
import { AppComponent } from './app.component';
Modules with javascript
What does a module written in javascript look like?
Let's take a very simple example of a JavaScript program.
To make it work we need two things
- A code editor (I recommend Visual Studio code)
- The Node.js javascript platform
To install these two tools you can follow this guide
https://www.ganatan.com/tutorials/getting-started-with-angular
For the exercise, just type the following code in an app.js file
function movieName(name) {
console.log('Movie name :' + name);
}
function movieDirector(director) {
console.log('Movie Director : ' + director);
}
function app() {
movieName('Gladiator');
movieDirector('Ridley Scott');
}
app();
To run it as it is a javascript file ( js extension ) type the following node command
- node app.js
Now some explanations.
The app function is called when executing the app.js script.
This function in turn calls the two functions movieName and movieDirector.
At this stage the code is simple and quite practical.
Everything is contained in one file and easy to read.
But obviously as modifications continue, the number of functions will probably increase.
From two functions we could end up calling hundreds of them.
And as always the day we are in a hurry it will be less easy to find our way!
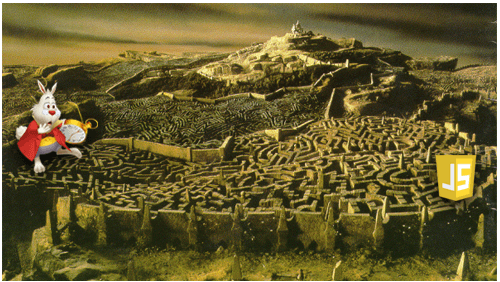
A javascript example with Modules
Let's now adapt our code to use this famous module system.
To do this, let's transform our app.js file into 3 separate files .
- app-modules.js
- movie-name.js
- movie-director.js
import { movieName } from './movie-name.js';
import { movieDirector } from './movie-director.js';
function app() {
movieName('Gladiator');
movieDirector('Ridley Scott');
}
app();
function movieName(name) {
console.log('Movie name :' + name);
}
export { movieName };
function movieDirector(director) {
console.log('Movie Director : ' + director)
}
export { movieDirector };
How to run a javascript example with Modules?
The program is better structured and it will be easier to make changes.
To finish this example the idea would be to execute the command
- node app-modules.js
But as is too often the case in IT, nothing works as we imagine.
The previous command will give the following error
- SyntaxError: Cannot use import statement outside a module
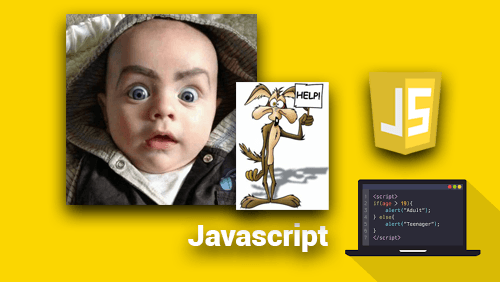
To make our test work, we will proceed as follows:
- Create an index.html file
- Call the script with the type="module" option
- And to refine everything, let's also add an icon file favicon.ico
Which will give the following file
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, user-scalable=no">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<title>Page Title</title>
</head>
<body>
<h1>Page content</h1>
<script type="module" src="app-modules.js"></script>
</body>
</html>
To finish one last mistake.
If you run index.html in your browser, you will get the following error.
Access to script at 'app-modules.js' from origin 'null' has been blocked by CORS policy
ES6 modules do not work locally.
So let's install an http server.
Node.js will allow us to do this and finally test our javascript program.
# Install an http server
npm install -g httpserver
# Run server, index.html file is used by default
http-server -o
# Test the app
http://localhost:8080/
If Chrome is your browser use F12 and the console tab to check the display.
- Movie name: Gladiator
- Movie Director: Ridley Scott
As they say “you have to see it to believe it”.
ES6 modules work.
Angular and ngModules
Whether with Javascript or with Angular the same question will arise.
The more the development of our application will advance, the more the functionalities will be numerous, and the more it will be difficult to navigate in the code.
Angular as a major framework allows division into modules.
The objective being to separate the different functionalities of the application in order to better organize our code.
Angular has its own module management system.
It is called NgModules.
Angular relies on both the ES6 module system as well as its own NgModules system .
We will use the official Angular documentation to understand Modules.
Useful addresses are as follows
- https://angular.io/guide/architecture-modules
- https://angular.io/guide/ngmodules
- https://angular.io/guide/ngmodule-vs-jsmodule
Angular therefore has its own module management system.
It is called NgModules.
Before going further, an image will allow us to describe a basic Angular application and the concept of modules.
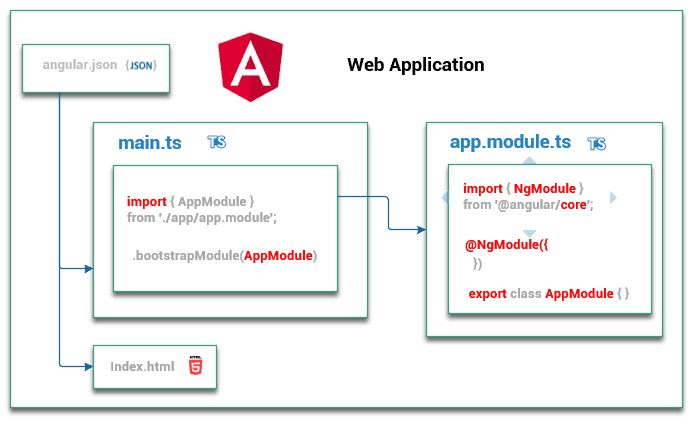
Let's proceed step by step.
Our base application has a single entry point.
The main.ts file
This file launches or Bootstrapping the root module.
This root module (translated into French as root) is called AppModule.
It is contained in the app.module.ts file
This AppModule module uses the Angular library @angular/core which allows it to use the NgModule keyword.
A few remarks to complete this
- Each Angular Application has at least one module .
- The "root module" is a classic module whose particularity is to define the "root component" of the application via the bootstrap property.
- NgModule is a typescript interface.
In the end we end up with the following source code
- app.module.ts
- main.ts
import { NgModule } from '@angular/core';
@NgModule({
declarations: [ ],
bootstrap: [AppComponent]
})
export class AppModule { }
import { AppModule } from './app/app.module';
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.error(err));
The concept of Angular Modules
Now that we have had an overview of Angular and its modules, let's go into details to understand this concept better.
Angular modules therefore represent an essential concept in the way this framework works.
They play a major role in structuring all Angular applications.
We start by talking about Angular Module.
- An Angular Module groups together within the same logical unit a certain number of Angular artifacts (or elements)
components, directives, pipes, services .... - An Angular Module defines the dependencies on other modules necessary for its own operation.
- An Angular module is simply defined with a class and the @NgModule decorator.
To clear things up, a representation of our first module.
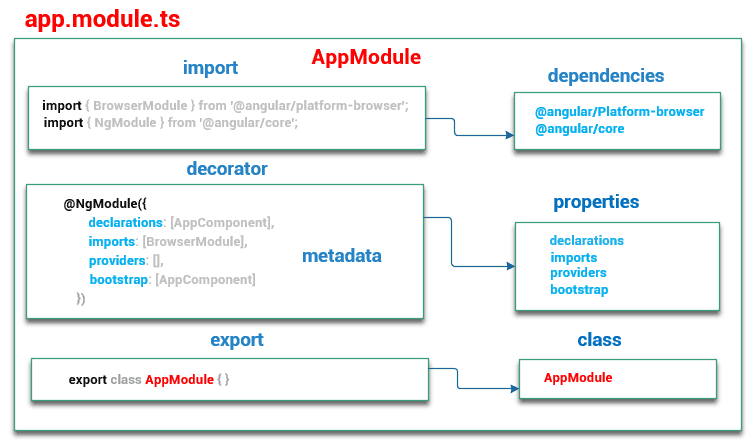
NgModule and decorator
An NgModule is a class described by a decorated with @NgModule().
@NgModule() is a function that has a metadata object
These properties describe the module
The @NgModule decorator will dynamically attach new responsibilities to this class.
It modifies the javascript class by providing metadata (or metadata ) to compose new functionalities.
This metadata will therefore allow us to define the responsibilities of the class.
This metadata has properties .
Below is a non-exhaustive list of its properties.
- statements
Defines the list of elements belonging to this module.
These will be for example directives, pipes, components....
- exports
Defines the list of components that will be visible and can therefore be used by modules that import the module.
Undeclared components will therefore not be exported and can only be used by the components contained in the module.
- imports
Defines the list of module dependencies, i.e. the modules on which our module depends.
- providers
This property allows you to declare the services that you will create as part of this module.
- bootstrap
Defines the root component which will contain all the other components of your application.
Only the root module can declare this property.
exports: The subset of declarations that should be visible and usable in the component templates of other NgModules.
imports: Other modules whose exported classes are needed by component templates declared in this NgModule.
providers: Creators of services that this NgModule contributes to the global collection of services; they become accessible in all parts of the app. (You can also specify providers at the component level, which is often preferred.)
bootstrap: The main application view, called the root component, which hosts all other app views. Only the root
Shared Module
Code factorization helps organize and streamline our code.
It allows you to bring together sequences of identical instructions scattered throughout a program into a single function.
It thus improves the readability of the code and facilitates correction and subsequent modifications.
Angular will allow us to apply factorization by creating shared modules .
Tips from the Angular team are at:
https://angular.io/guide/sharing-ngmodules
Shared modules will allow us to share directives, pipes, and components in a single module.
It will then suffice to import this module into any part of our application where we need it.
Angular CLI and modules
Angular CLI stands for Angular Command Line Interface.
Angular CLI is probably the most important tool provided by the Angular Framework.
It will allow you to initialize, develop and maintain your Angular applications.
The commands provided by this tool automatically manage declarations, imports, or bootstrap.
Angular CLI therefore implicitly adds module management when creating your code.
We now have to experiment with the modules.
Since we're not going to reinvent the wheel every time
We will obviously use Angular CLI, it was made for that.
As the exercises progress, we will see how the principles of the modules are implemented.
This tutorial offers you two scenarios
- A basic application created from scratch
- A prototype application based on Angular CLI , Routing , Lazy loading and the Bootstrap Framework
So without further ado, we must get down to practice.
Choose your weapons and battle stations.
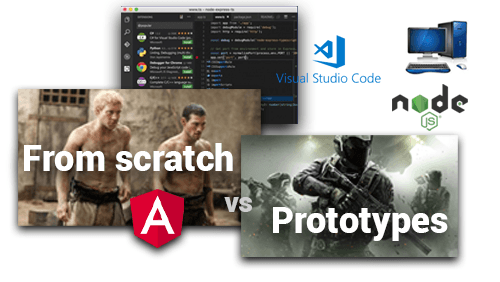
Application From scratch
So let's create our basic Angular application.
From Scratch meaning we will create it from scratch with no outside source code.
We couldn't be more educational!
As we saw before we will only need two tools
- Node.js
- A code editor
We just have to execute the following commands.
# Installing angular-cli latest version available
npm install -g @angular/cli
# Installed version test
ng --version
# Generate a project arbitrarily called angular-starter with default options
ng new angular-starter --defaults
# Position yourself in the project
cd angular-starter
# Execute
ng serve
This is why we can qualify Angular as a Framework .
A few command lines and the application magically appears.
It's " all in one ". And quality too!
Now let's analyze the source code generated by Angular CLI.
We will try to find all the elements related to the modules that we have mentioned so far.
Let's start with the app.module.ts file
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Let's describe step by step what angular CLI did.
How to create a module?
- export class AppModule
- The module is a class
- It is called AppModule
- It is exported and can therefore be used by other modules
How to describe the characteristics of this module?
- @NgModule ({ .... })
The essential element is the decorator .
The @ character symbolizes the decorator.
The decorator is a design pattern.
It describes the broad outlines of a solution.
Prototype Application
Now let's use a more robust application containing a certain number of functionalities.
To be able to continue this tutorial we need to add a tool
- Git : Version management software.
We are going to use an existing project whose characteristics are
- Generated with Angular CLI
- Routing
- Lazy-loading
- Using the Bootstrap CSS Framework
The commands to use are as follows
# Create a demo directory (the name here is arbitrary)
mkdir demo
# Go to this directory
cd demo
# Get the source code on your workstation
git clone https://github.com/ganatan/angular-react-bootstrap
# Go to the directory that was created
cd angular-react-bootstrap
cd angular
# Run the installation of dependencies (or libraries)
npm install
# Run the program
npm run start
# Check how it works by running the command in your browser
http://localhost:4200/
Tests
All that remains is to test the different Angular scripts.
# Développement
npm run start
http://localhost:4200/
# Tests
npm run lint
npm run test
# Compilation
npm run build
Source Code
By following each of the tips I've given you in this guide, you end up with an Angular source code.
The source code obtained at the end of this tutorial is available on github
https://github.com/ganatan/angular-react-modules
The following steps will get you a prototype application .
- Step 6: Server Side Rendering with Angular
- Step 7: Progressive Web App with Angular
- Step 8: Search Engine Optimization with Angular
- Step 9: HttpClient with Angular
This last step provides an example of an application
The source code of this final application is available on GitHub
https://github.com/ganatan/angular-app